$query = new EntityFieldQuery();
$query->entityCondition('entity_type', 'node')
->entityCondition('bundle', 'webform');
$result = $query->execute();
$options = array(
0 => 'None'
);
if (!empty($result['node'])) {
$nids = array_keys($result['node']);
$nodes = node_load_multiple($nids);
foreach ($nodes as $nid => $node) {
$options[$nid] = $node->label();
}
}
return $options;
I use the above code to retrieve nodes that are webforms.
What I would like to know is what condition to use to get only the webforms that have the block functionality?
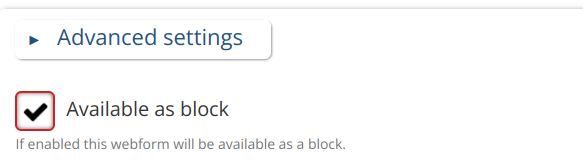
The actual query would look like this (untested):
That will fetch an array of node IDs.