Hello everyone, I have a question about creating nodes in Backdrop CMS. Currently, users need to access the node creation form to publish content, but I would like to know if there is an option or module that allows creating a node directly from a form embedded in a block, without needing to access the standard form.
I have explored some alternatives:
- Webform: I couldn’t find a module that allows creating nodes directly through a Webform.
- Rules: I tried using Rules to achieve this, but I encountered an error because the Webform Rules module is obsolete and not compatible with my version of Backdrop CMS.
- formblock: Update 11 years ago
Is there a recommended module or method to accomplish this? I would appreciate any suggestions or examples of how to implement it.
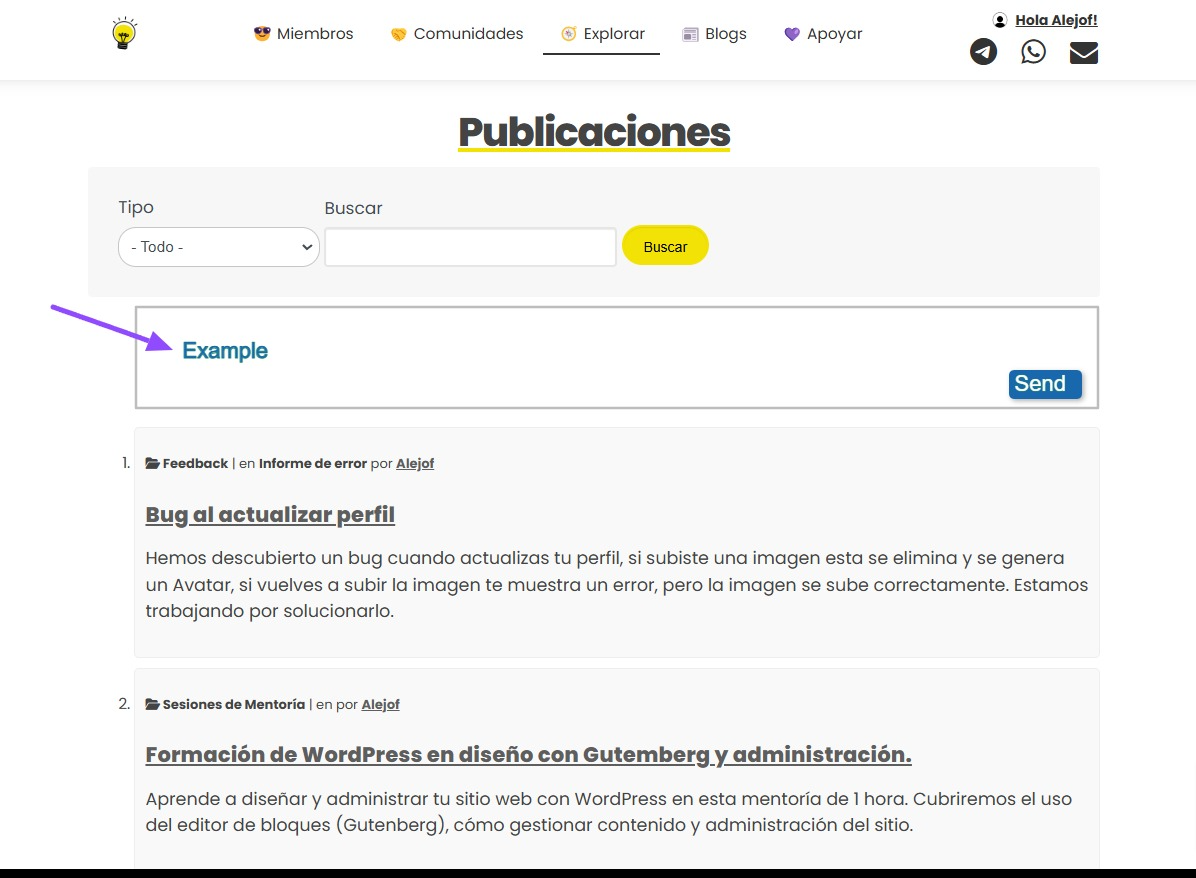
There is a work in progress at https://github.com/backdrop-contrib/webformtonode but I don't its ready yet.